Account
ArmsysApi 0.12.18 |
#include <BUsbSerial.h>
#include <BSys.h>
#include <bstdio.h>
#include <BDebug.h>
#include <usbd_conf.h>
#include <usbd_def.h>
#include <usbd_ioreq.h>
Macros | |
#define | L1DEBUG 0 |
#define | L2DEBUG 0 |
#define | L3DEBUG 0 |
#define | dl1printf(fmt, a...) |
#define | dl2printf(fmt, a...) |
#define | dl3printf(fmt, a...) |
#define | USBD_VID busbConfig.vendorId |
#define | USBD_PID busbConfig.productId |
#define | USBD_LANGID_STRING 0x409 |
#define | USBD_MANUFACTURER_STRING busbConfig.manufacturer |
#define | USBD_PRODUCT_HS_STRING busbConfig.product |
#define | USBD_SERIALNUMBER_HS_STRING busbConfig.serialNumber |
#define | USBD_PRODUCT_FS_STRING busbConfig.product |
#define | USBD_SERIALNUMBER_FS_STRING busbConfig.serialNumber |
#define | USBD_CONFIGURATION_HS_STRING "VCP Config" |
#define | USBD_INTERFACE_HS_STRING "VCP Interface" |
#define | USBD_CONFIGURATION_FS_STRING "VCP Config" |
#define | USBD_INTERFACE_FS_STRING "VCP Interface" |
Functions | |
BUInt8 * | USBD_VCP_DeviceDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_LangIDStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_ProductStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_ManufacturerStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_SerialStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_ConfigStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
BUInt8 * | USBD_VCP_InterfaceStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
void | HAL_PCD_SetupStageCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_DataOutStageCallback (PCD_HandleTypeDef *hpcd, BUInt8 epnum) |
void | HAL_PCD_DataInStageCallback (PCD_HandleTypeDef *hpcd, BUInt8 epnum) |
void | HAL_PCD_SOFCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_ResetCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_SuspendCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_ResumeCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_ISOOUTIncompleteCallback (PCD_HandleTypeDef *hpcd, BUInt8 epnum) |
void | HAL_PCD_ISOINIncompleteCallback (PCD_HandleTypeDef *hpcd, BUInt8 epnum) |
void | HAL_PCD_ConnectCallback (PCD_HandleTypeDef *hpcd) |
void | HAL_PCD_DisconnectCallback (PCD_HandleTypeDef *hpcd) |
USBD_StatusTypeDef | USBD_LL_DeInit (USBD_HandleTypeDef *pdev) |
USBD_StatusTypeDef | USBD_LL_Start (USBD_HandleTypeDef *pdev) |
USBD_StatusTypeDef | USBD_LL_Stop (USBD_HandleTypeDef *pdev) |
USBD_StatusTypeDef | USBD_LL_OpenEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr, BUInt8 ep_type, uint16_t ep_mps) |
USBD_StatusTypeDef | USBD_LL_CloseEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
USBD_StatusTypeDef | USBD_LL_FlushEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
USBD_StatusTypeDef | USBD_LL_StallEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
USBD_StatusTypeDef | USBD_LL_ClearStallEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
BUInt8 | USBD_LL_IsStallEP (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
USBD_StatusTypeDef | USBD_LL_SetUSBAddress (USBD_HandleTypeDef *pdev, BUInt8 dev_addr) |
USBD_StatusTypeDef | USBD_LL_Transmit (USBD_HandleTypeDef *pdev, BUInt8 ep_addr, BUInt8 *pbuf, uint16_t size) |
USBD_StatusTypeDef | USBD_LL_PrepareReceive (USBD_HandleTypeDef *pdev, BUInt8 ep_addr, BUInt8 *pbuf, uint16_t size) |
uint32_t | USBD_LL_GetRxDataSize (USBD_HandleTypeDef *pdev, BUInt8 ep_addr) |
void | USBD_LL_Delay (uint32_t Delay) |
USBD_StatusTypeDef | USBD_LL_Init (USBD_HandleTypeDef *pdev) |
void | OTG_FS_IRQHandler (void) |
void | OTG_HS_IRQHandler (void) |
static BUInt8 | usbdInit (USBD_HandleTypeDef *pdev, BUInt8 cfgidx) |
static BUInt8 | usbdDeInit (USBD_HandleTypeDef *pdev, BUInt8 cfgidx) |
static BUInt8 | usbdSetup (USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req) |
static BUInt8 | usbdRx0 (USBD_HandleTypeDef *pdev) |
static BUInt8 | usbdRx (USBD_HandleTypeDef *pdev, BUInt8 epnum) |
static BUInt8 | usbdTxSent (USBD_HandleTypeDef *pdev, BUInt8 epnum) |
static BUInt8 * | usbdGetHSCfgDesc (uint16_t *length) |
static BUInt8 * | usbdGetFSCfgDesc (uint16_t *length) |
static BUInt8 * | usbdGetOtherSpeedCfgDesc (uint16_t *length) |
static BUInt8 * | usbdGetDeviceQualifierDescriptor (uint16_t *length) |
static int | usbWrite (const char *data, int nBytes) |
Variables | |
const int | PacketSizeHighSpeed = 512 |
const int | PacketSizeFullSpeed = 64 |
const int | PacketSizeCmd = 8 |
const int | ConfigDescriptorSize = 67 |
const int | EndPointCmd = 0x82 |
const int | EndPointTx = 0x81 |
const int | EndPointRx = 0x01 |
static BUsbConfig | busbConfig |
__ALIGN_BEGIN BUInt8 hUSBDDeviceDesc [USB_LEN_DEV_DESC] | __ALIGN_END |
USBD_DescriptorsTypeDef | VCP_Desc |
static BUsbSerial * | usbSerial |
USBD_ClassTypeDef | usbdClass |
Macro Definition Documentation
◆ dl1printf
#define dl1printf | ( | fmt, | |
a... | |||
) |
◆ dl2printf
#define dl2printf | ( | fmt, | |
a... | |||
) |
◆ dl3printf
#define dl3printf | ( | fmt, | |
a... | |||
) |
◆ L1DEBUG
#define L1DEBUG 0 |
◆ L2DEBUG
#define L2DEBUG 0 |
◆ L3DEBUG
#define L3DEBUG 0 |
◆ USBD_CONFIGURATION_FS_STRING
#define USBD_CONFIGURATION_FS_STRING "VCP Config" |
◆ USBD_CONFIGURATION_HS_STRING
#define USBD_CONFIGURATION_HS_STRING "VCP Config" |
◆ USBD_INTERFACE_FS_STRING
#define USBD_INTERFACE_FS_STRING "VCP Interface" |
◆ USBD_INTERFACE_HS_STRING
#define USBD_INTERFACE_HS_STRING "VCP Interface" |
◆ USBD_LANGID_STRING
#define USBD_LANGID_STRING 0x409 |
◆ USBD_MANUFACTURER_STRING
#define USBD_MANUFACTURER_STRING busbConfig.manufacturer |
◆ USBD_PID
#define USBD_PID busbConfig.productId |
◆ USBD_PRODUCT_FS_STRING
#define USBD_PRODUCT_FS_STRING busbConfig.product |
◆ USBD_PRODUCT_HS_STRING
#define USBD_PRODUCT_HS_STRING busbConfig.product |
◆ USBD_SERIALNUMBER_FS_STRING
#define USBD_SERIALNUMBER_FS_STRING busbConfig.serialNumber |
◆ USBD_SERIALNUMBER_HS_STRING
#define USBD_SERIALNUMBER_HS_STRING busbConfig.serialNumber |
◆ USBD_VID
#define USBD_VID busbConfig.vendorId |
Function Documentation
◆ HAL_PCD_ConnectCallback()
void HAL_PCD_ConnectCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_DataInStageCallback()
void HAL_PCD_DataInStageCallback | ( | PCD_HandleTypeDef * | hpcd, |
BUInt8 | epnum | ||
) |
◆ HAL_PCD_DataOutStageCallback()
void HAL_PCD_DataOutStageCallback | ( | PCD_HandleTypeDef * | hpcd, |
BUInt8 | epnum | ||
) |
◆ HAL_PCD_DisconnectCallback()
void HAL_PCD_DisconnectCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_ISOINIncompleteCallback()
void HAL_PCD_ISOINIncompleteCallback | ( | PCD_HandleTypeDef * | hpcd, |
BUInt8 | epnum | ||
) |
◆ HAL_PCD_ISOOUTIncompleteCallback()
void HAL_PCD_ISOOUTIncompleteCallback | ( | PCD_HandleTypeDef * | hpcd, |
BUInt8 | epnum | ||
) |
◆ HAL_PCD_ResetCallback()
void HAL_PCD_ResetCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_ResumeCallback()
void HAL_PCD_ResumeCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_SetupStageCallback()
void HAL_PCD_SetupStageCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_SOFCallback()
void HAL_PCD_SOFCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ HAL_PCD_SuspendCallback()
void HAL_PCD_SuspendCallback | ( | PCD_HandleTypeDef * | hpcd | ) |
◆ OTG_FS_IRQHandler()
void OTG_FS_IRQHandler | ( | void | ) |
◆ OTG_HS_IRQHandler()
void OTG_HS_IRQHandler | ( | void | ) |
◆ USBD_LL_ClearStallEP()
USBD_StatusTypeDef USBD_LL_ClearStallEP | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr | ||
) |
◆ USBD_LL_CloseEP()
USBD_StatusTypeDef USBD_LL_CloseEP | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr | ||
) |
◆ USBD_LL_DeInit()
USBD_StatusTypeDef USBD_LL_DeInit | ( | USBD_HandleTypeDef * | pdev | ) |
◆ USBD_LL_Delay()
void USBD_LL_Delay | ( | uint32_t | Delay | ) |
◆ USBD_LL_FlushEP()
USBD_StatusTypeDef USBD_LL_FlushEP | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr | ||
) |
◆ USBD_LL_GetRxDataSize()
uint32_t USBD_LL_GetRxDataSize | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr | ||
) |
◆ USBD_LL_Init()
USBD_StatusTypeDef USBD_LL_Init | ( | USBD_HandleTypeDef * | pdev | ) |
◆ USBD_LL_IsStallEP()
◆ USBD_LL_OpenEP()
USBD_StatusTypeDef USBD_LL_OpenEP | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr, | ||
BUInt8 | ep_type, | ||
uint16_t | ep_mps | ||
) |
◆ USBD_LL_PrepareReceive()
USBD_StatusTypeDef USBD_LL_PrepareReceive | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr, | ||
BUInt8 * | pbuf, | ||
uint16_t | size | ||
) |
◆ USBD_LL_SetUSBAddress()
USBD_StatusTypeDef USBD_LL_SetUSBAddress | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | dev_addr | ||
) |
◆ USBD_LL_StallEP()
USBD_StatusTypeDef USBD_LL_StallEP | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr | ||
) |
◆ USBD_LL_Start()
USBD_StatusTypeDef USBD_LL_Start | ( | USBD_HandleTypeDef * | pdev | ) |
◆ USBD_LL_Stop()
USBD_StatusTypeDef USBD_LL_Stop | ( | USBD_HandleTypeDef * | pdev | ) |
◆ USBD_LL_Transmit()
USBD_StatusTypeDef USBD_LL_Transmit | ( | USBD_HandleTypeDef * | pdev, |
BUInt8 | ep_addr, | ||
BUInt8 * | pbuf, | ||
uint16_t | size | ||
) |
◆ USBD_VCP_ConfigStrDescriptor()
BUInt8* USBD_VCP_ConfigStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_DeviceDescriptor()
BUInt8* USBD_VCP_DeviceDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_InterfaceStrDescriptor()
BUInt8* USBD_VCP_InterfaceStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_LangIDStrDescriptor()
BUInt8* USBD_VCP_LangIDStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_ManufacturerStrDescriptor()
BUInt8* USBD_VCP_ManufacturerStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_ProductStrDescriptor()
BUInt8* USBD_VCP_ProductStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ USBD_VCP_SerialStrDescriptor()
BUInt8* USBD_VCP_SerialStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
◆ usbdDeInit()
◆ usbdGetDeviceQualifierDescriptor()
| static |
◆ usbdGetFSCfgDesc()
| static |
◆ usbdGetHSCfgDesc()
| static |
◆ usbdGetOtherSpeedCfgDesc()
| static |
◆ usbdInit()
◆ usbdRx()
◆ usbdRx0()
| static |
◆ usbdSetup()
| static |
◆ usbdTxSent()
◆ usbWrite()
| static |
Variable Documentation
◆ __ALIGN_END
__ALIGN_BEGIN BUInt8 usbdDeviceQualifierDesc [USB_LEN_DEV_QUALIFIER_DESC] __ALIGN_END |
◆ busbConfig
| static |
Initial value:
= {
0x0483,
0x5740,
0x00,
0x00,
0x00,
"Beam",
"Armsys",
"0",
}
◆ ConfigDescriptorSize
const int ConfigDescriptorSize = 67 |
◆ EndPointCmd
const int EndPointCmd = 0x82 |
◆ EndPointRx
const int EndPointRx = 0x01 |
◆ EndPointTx
const int EndPointTx = 0x81 |
◆ PacketSizeCmd
const int PacketSizeCmd = 8 |
◆ PacketSizeFullSpeed
const int PacketSizeFullSpeed = 64 |
◆ PacketSizeHighSpeed
const int PacketSizeHighSpeed = 512 |
◆ usbdClass
USBD_ClassTypeDef usbdClass |
Initial value:
= {
NULL,
NULL,
NULL,
NULL,
}
static BUInt8 * usbdGetDeviceQualifierDescriptor(uint16_t *length)
Definition: BUsbSerial.cpp:722
static BUInt8 usbdInit(USBD_HandleTypeDef *pdev, BUInt8 cfgidx)
Definition: BUsbSerial.cpp:674
static BUInt8 usbdTxSent(USBD_HandleTypeDef *pdev, BUInt8 epnum)
Definition: BUsbSerial.cpp:696
static BUInt8 usbdDeInit(USBD_HandleTypeDef *pdev, BUInt8 cfgidx)
Definition: BUsbSerial.cpp:678
static BUInt8 * usbdGetOtherSpeedCfgDesc(uint16_t *length)
Definition: BUsbSerial.cpp:717
static BUInt8 usbdSetup(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req)
Definition: BUsbSerial.cpp:682
◆ usbSerial
| static |
◆ VCP_Desc
USBD_DescriptorsTypeDef VCP_Desc |
Initial value:
= {
}
BUInt8 * USBD_VCP_ConfigStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:150
BUInt8 * USBD_VCP_InterfaceStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:160
BUInt8 * USBD_VCP_ManufacturerStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:135
BUInt8 * USBD_VCP_ProductStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:125
BUInt8 * USBD_VCP_LangIDStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:120
BUInt8 * USBD_VCP_SerialStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:140
BUInt8 * USBD_VCP_DeviceDescriptor(USBD_SpeedTypeDef speed, uint16_t *length)
Definition: BUsbSerial.cpp:115
Generated by
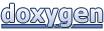