Account
ArmsysApi 0.12.18 |
#include <BUsbSerial.h>
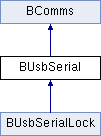
Public Member Functions | |
BUsbSerial (BUInt rxFifoSize=512, BUInt txFifoSize=512) | |
BError | init (BUsbType type=BUsbTypeFullSpeed, BUsbConfig *usbConfig=0) |
Initialise with optional config params. More... | |
void | setMaximumUsbChunk (BUInt usbChunkSize) |
Sets the maximum USB tranmitted chunk size. More... | |
void | close () |
Close down the USB interface. More... | |
void | setBlocking (Bool on) |
Enable blocking mode. More... | |
void | setSync (Bool on) |
Set synchronous mode for writes. More... | |
void | start () |
Start processing. More... | |
int | printf (const char *fmt,...) |
int | printfv (const char *fmt, va_list args) |
BUInt | writeAvailable () |
How much write space is available. More... | |
BError | write (const void *data, BUInt32 nBytes, BUInt32 &nWritten) |
Write a set of data. More... | |
BUInt | readAvailable () |
How many bytes of read data is available. More... | |
BError | read (void *data, BUInt32 nBytes, BUInt32 &nRead) |
Read the data. More... | |
BError | wait (BUInt32 eventSet, BTimeout timeoutUs=BTimeoutForever, BUInt32 num=1) |
BError | usbdInitHardware () |
BError | usbdInitUsbDev (USBD_HandleTypeDef *pdev) |
BUInt8 | usbdInit (USBD_HandleTypeDef *pdev, BUInt8 cfgidx) |
void | usbdStarted () |
The USB device is connected and running. More... | |
BUInt8 | usbdDeInit (USBD_HandleTypeDef *pdev, BUInt8 cfgidx) |
BUInt8 | usbdSetup (USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req) |
void | usbdTx () |
Data to USB stack. More... | |
BUInt8 | usbdTxComplete () |
Data has been sent. More... | |
int | usbdRx (USBD_HandleTypeDef *pdev, BUInt len) |
Data from USB stack. More... | |
void | usbdInterrupt () |
![]() | |
BComms () | |
virtual | ~BComms () |
virtual BError | init () |
virtual const char * | name () |
The name of this interface. More... | |
virtual BUInt32 | byteRate () |
The byte rate of this interface. More... | |
virtual BError | setPacketMode (Bool packetMode) |
Set packet mode. More... | |
virtual Bool | packetMode () |
Device is in packet mode. More... | |
virtual BError | setTimeout (BTimeout timeoutUs) |
Set communication timeout. More... | |
virtual BError | connect (const char *resource) |
Create a connection. More... | |
virtual Bool | isConnected () |
virtual BError | disconnect () |
Disconnect. More... | |
virtual void | flush (Flush flush) |
virtual BError | writeChunks (const BDataChunk *chunks, BUInt nChunks, BUInt32 &nTrans) |
virtual void | eventQueue (BEventQueue *eventQueue, BUInt32 event, BUInt32 eventSet, BUInt num=1) |
virtual void | eventEnable (Bool on) |
Enable events to be sent. More... | |
Private Attributes | |
BFifo< char > | otxFifo |
The transmit fifo. More... | |
BFifo< char > | orxFifo |
The receive fifo. More... | |
Bool | ohighSpeed |
High speed USB. More... | |
BUInt | opacketSize |
The maximum packet size. More... | |
Bool | oinitialised |
The USB device has initialised. More... | |
Bool | oconnected |
The USB is connected. More... | |
BSemaphore | owait |
Read wait semaphore. More... | |
BUInt | owaitReadNum |
The number of bytes to wait for. More... | |
Bool | oblocking |
Device set to block on writes. More... | |
Bool | osync |
Device set to wait for writes. More... | |
USBD_HandleTypeDef | ousbDev |
The USB device. More... | |
PCD_HandleTypeDef | ohpcd |
The USB PCD layer. More... | |
Bool | otxSending |
USB is transmitting. More... | |
int | otxSendLen |
The length of data sent. More... | |
char | orxBuffer [512] |
Receive packet buffer. More... | |
Bool | orxStalled |
Receive has stalled as no room in buffer. More... | |
BUInt | ousbChunkSize |
The maximum USB chunk size. More... | |
Additional Inherited Members | |
![]() | |
enum | Flush { FlushRead, FlushWrite, FlushReadWrite } |
![]() | |
Bool | oconnected |
Bool | opacketMode |
BTimeout | otimeout |
BEventQueue * | oeventQueue |
Bool | oeventEnabled |
BUInt32 | oevent |
BUInt32 | oeventSet |
BUInt | oeventNum |
Constructor & Destructor Documentation
◆ BUsbSerial()
Member Function Documentation
◆ close()
| virtual |
Close down the USB interface.
Reimplemented from BComms.
◆ init()
BError BUsbSerial::init | ( | BUsbType | type = BUsbTypeFullSpeed , |
BUsbConfig * | usbConfig = 0 | ||
) |
Initialise with optional config params.
◆ printf()
int BUsbSerial::printf | ( | const char * | fmt, |
... | |||
) |
◆ printfv()
int BUsbSerial::printfv | ( | const char * | fmt, |
va_list | args | ||
) |
◆ read()
◆ readAvailable()
| virtual |
How many bytes of read data is available.
Reimplemented from BComms.
Reimplemented in BUsbSerialLock.
◆ setBlocking()
void BUsbSerial::setBlocking | ( | Bool | on | ) |
Enable blocking mode.
◆ setMaximumUsbChunk()
void BUsbSerial::setMaximumUsbChunk | ( | BUInt | usbChunkSize | ) |
Sets the maximum USB tranmitted chunk size.
◆ setSync()
void BUsbSerial::setSync | ( | Bool | on | ) |
Set synchronous mode for writes.
◆ start()
void BUsbSerial::start | ( | ) |
Start processing.
◆ usbdDeInit()
◆ usbdInit()
◆ usbdInitHardware()
BError BUsbSerial::usbdInitHardware | ( | ) |
◆ usbdInitUsbDev()
BError BUsbSerial::usbdInitUsbDev | ( | USBD_HandleTypeDef * | pdev | ) |
◆ usbdInterrupt()
void BUsbSerial::usbdInterrupt | ( | void | ) |
◆ usbdRx()
int BUsbSerial::usbdRx | ( | USBD_HandleTypeDef * | pdev, |
BUInt | len | ||
) |
Data from USB stack.
◆ usbdSetup()
BUInt8 BUsbSerial::usbdSetup | ( | USBD_HandleTypeDef * | pdev, |
USBD_SetupReqTypedef * | req | ||
) |
◆ usbdStarted()
void BUsbSerial::usbdStarted | ( | ) |
The USB device is connected and running.
◆ usbdTx()
void BUsbSerial::usbdTx | ( | ) |
Data to USB stack.
◆ usbdTxComplete()
BUInt8 BUsbSerial::usbdTxComplete | ( | ) |
Data has been sent.
◆ wait()
| virtual |
Reimplemented from BComms.
◆ write()
◆ writeAvailable()
| virtual |
Member Data Documentation
◆ oblocking
| private |
Device set to block on writes.
◆ oconnected
| private |
The USB is connected.
◆ ohighSpeed
| private |
High speed USB.
◆ ohpcd
| private |
The USB PCD layer.
◆ oinitialised
| private |
The USB device has initialised.
◆ opacketSize
| private |
The maximum packet size.
◆ orxBuffer
| private |
Receive packet buffer.
◆ orxFifo
| private |
The receive fifo.
◆ orxStalled
| private |
Receive has stalled as no room in buffer.
◆ osync
| private |
Device set to wait for writes.
◆ otxFifo
| private |
The transmit fifo.
◆ otxSending
| private |
USB is transmitting.
◆ otxSendLen
| private |
The length of data sent.
◆ ousbChunkSize
| private |
The maximum USB chunk size.
◆ ousbDev
| private |
The USB device.
◆ owait
| private |
Read wait semaphore.
◆ owaitReadNum
| private |
The number of bytes to wait for.
The documentation for this class was generated from the following files:
Generated by
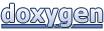