Account
ArmsysApi 0.12.18 |
Public Types | Public Member Functions | Protected Member Functions | Protected Attributes | List of all members
BHttpServer Class Reference
#include <BHttpServer.h>
Inheritance diagram for BHttpServer:

Public Types | |
enum | ReadType { ReadTypeData, ReadTypeLine, ReadTypeDataLine } |
Public Member Functions | |
BHttpServer (BUInt rxFifoSize=1500, BUInt txFifoSize=1500) | |
BError | init () |
void | run () |
The tasks main run loop. More... | |
virtual BError | processUrl () |
virtual BError | processHome () |
virtual BError | pageSend (const char *title, const char *str) |
virtual BError | pageSendError (const char *str, const char *status="403 Error") |
virtual BError | pageSendHead (const char *title) |
virtual BError | pageSendTail () |
void | cookieSet (BString name, BString value) |
BString | cookieGet (BString name) |
void | writeTableRow (const char *name, const char *title, const char *value, const char *units=0, int errNum=0) |
void | writeTableInput (const char *name, const char *title, const char *type, const char *value, const char **args=0) |
void | writeTableInput (const char *name, const char *title, const char *type, const char *value, BString str) |
BError | writeHead (const char *status, const char *contentType, BUInt size=0, Bool cache=0, Bool chunked=0, BStringList extra=BStringList()) |
BError | writeString (const char *str) |
BError | writeChunk (const void *data, int len) |
BError | writeFlush () |
BError | writeTail () |
virtual BError | setHeader (const char *status, const char *contentType, BUInt size=0, Bool cache=0, Bool chunked=0, BStringList extra=BStringList()) |
virtual BError | processRequest () |
BError | readData (BUInt len, BUInt32 &nt, ReadType readType) |
![]() | |
BTask (const char *name="", BUInt stackSize=1024, BUInt priority=1) | |
~BTask () | |
void | init (const char *name, BUInt stackSize=1024, BUInt priority=1) |
BError | start () |
Starts the task running. More... | |
void | stop () |
Stops the task. More... | |
void | setPriority (BUInt priority) |
Set the priority of the task: 0 upwards. More... | |
void | delayMs (BUInt ms) |
Delay for a time in ms. More... | |
Protected Member Functions | |
void * | function () |
virtual BError | devInit () |
virtual void | devRun () |
virtual BError | devWrite (const void *data, BUInt len, BUInt32 &nt) |
virtual BError | devProcess (Bool wait) |
Protected Attributes | |
BFifoChar | orxFifo |
BFifoChar | otxFifo |
char * | obuffer |
BUInt | obufferNext |
BString | ocmd |
BString | ourl |
BString | oget |
BUInt | ocontentLen |
BString | ocontentTypeRecv |
BString | ocontentTypeSend |
BString | oboundary |
BString | oauthorisation |
BDictString | ocookies |
BDictString | ocookiesSend |
const char * | ohead_status |
const char * | ohead_contentType |
BUInt | ohead_size |
Bool | ohead_cache |
Bool | ohead_chunked |
BStringList | ohead_extra |
struct netconn * | osocketListen |
struct netconn * | osocket |
![]() | |
const char * | oname |
BUInt | ostackSize |
BUInt | opriority |
TaskHandle_t | otask |
Bool | orunning |
Additional Inherited Members | |
![]() | |
static void | runTasks () |
Main run tasks loop. More... | |
Member Enumeration Documentation
◆ ReadType
Constructor & Destructor Documentation
◆ BHttpServer()
Member Function Documentation
◆ cookieGet()
◆ cookieSet()
◆ devInit()
| protectedvirtual |
◆ devProcess()
◆ devRun()
| protectedvirtual |
◆ devWrite()
◆ function()
| protected |
◆ init()
BError BHttpServer::init | ( | ) |
◆ pageSend()
| virtual |
◆ pageSendError()
| virtual |
◆ pageSendHead()
| virtual |
◆ pageSendTail()
| virtual |
◆ processHome()
| virtual |
◆ processRequest()
| virtual |
◆ processUrl()
| virtual |
◆ readData()
◆ run()
| virtual |
The tasks main run loop.
Reimplemented from BTask.
◆ setHeader()
| virtual |
◆ writeChunk()
BError BHttpServer::writeChunk | ( | const void * | data, |
int | len | ||
) |
◆ writeFlush()
BError BHttpServer::writeFlush | ( | ) |
◆ writeHead()
BError BHttpServer::writeHead | ( | const char * | status, |
const char * | contentType, | ||
BUInt | size = 0 , | ||
Bool | cache = 0 , | ||
Bool | chunked = 0 , | ||
BStringList | extra = BStringList() | ||
) |
◆ writeString()
BError BHttpServer::writeString | ( | const char * | str | ) |
◆ writeTableInput() [1/2]
void BHttpServer::writeTableInput | ( | const char * | name, |
const char * | title, | ||
const char * | type, | ||
const char * | value, | ||
const char ** | args = 0 | ||
) |
◆ writeTableInput() [2/2]
void BHttpServer::writeTableInput | ( | const char * | name, |
const char * | title, | ||
const char * | type, | ||
const char * | value, | ||
BString | str | ||
) |
◆ writeTableRow()
void BHttpServer::writeTableRow | ( | const char * | name, |
const char * | title, | ||
const char * | value, | ||
const char * | units = 0 , | ||
int | errNum = 0 | ||
) |
◆ writeTail()
BError BHttpServer::writeTail | ( | ) |
Member Data Documentation
◆ oauthorisation
| protected |
◆ oboundary
| protected |
◆ obuffer
| protected |
◆ obufferNext
| protected |
◆ ocmd
| protected |
◆ ocontentLen
| protected |
◆ ocontentTypeRecv
| protected |
◆ ocontentTypeSend
| protected |
◆ ocookies
| protected |
◆ ocookiesSend
| protected |
◆ oget
| protected |
◆ ohead_cache
| protected |
◆ ohead_chunked
| protected |
◆ ohead_contentType
| protected |
◆ ohead_extra
| protected |
◆ ohead_size
| protected |
◆ ohead_status
| protected |
◆ orxFifo
| protected |
◆ osocket
| protected |
◆ osocketListen
| protected |
◆ otxFifo
| protected |
◆ ourl
| protected |
The documentation for this class was generated from the following files:
Generated by
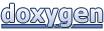