Account
ArmsysApi 0.12.18 |
#include <BString.h>
Public Member Functions | |
BString (const char *str=0, int len=-1, Bool copy=0) | |
BString (const BString &string) | |
BString (BChar c) | |
BString (BInt v) | |
~BString () | |
BString | copy () const |
Return an independant copy. More... | |
BUInt | len () const |
Length of string. More... | |
const char * | str () const |
Ptr to char* representation. More... | |
const char * | retStr () const |
Ptr to char* representation. More... | |
BInt | retInt () const |
Return string as a int. More... | |
BUInt | retUInt () const |
Return string as a int. More... | |
BFloat64 | retFloat64 () const |
Return string as a double. More... | |
int | compare (const BString &string) const |
Compare strings. More... | |
int | compare (const char *string) const |
Compare strings. More... | |
int | find (char ch) const |
Find ch in string searching forwards. More... | |
int | find (BString str) const |
Find string in string searching forwards. More... | |
int | findReverse (char ch) const |
Find ch in string searching backwards. More... | |
void | clear () |
Clear the string. More... | |
BString | add (const BString &str) const |
Add strings returning result. More... | |
BString & | truncate (BUInt len) |
Truncate to length len. More... | |
BString & | pad (BUInt len) |
Pad to length len. More... | |
BString & | printf (const char *fmt,...) |
Formated print into the string. More... | |
BString & | toUpper () |
Convert to uppercase. More... | |
BString & | toLower () |
Convert to lowercase. More... | |
BString | subString (BUInt start, int len) const |
Returns substring. More... | |
BString | reverse () const |
Reverse character order. More... | |
void | del (BUInt start, int len) |
Delete substring. More... | |
void | insert (BUInt start, const BString &str) |
Insert substring. More... | |
void | append (const BString &str) |
Append a string. More... | |
BUInt32 | hash () const |
retunr a hash value More... | |
BString | pullToken (const BString &terminators) |
Pull token from start of string. More... | |
BString | removeSeparators (BString separators) const |
Remove any char from sepatators from string. More... | |
BList< BString > | split (char splitChar) |
Split string into an array based on the character separator. More... | |
BString & | operator= (const BString &string) |
char & | operator[] (int pos) |
operator const char * () const | |
int | operator== (const BString &s) const |
int | operator== (const char *s) const |
int | operator!= (const BString &s) const |
int | operator!= (const char *s) const |
int | operator> (const BString &s) const |
int | operator> (const char *s) const |
int | operator< (const BString &s) const |
int | operator< (const char *s) const |
int | operator>= (const BString &s) const |
int | operator>= (const char *s) const |
int | operator<= (const BString &s) const |
int | operator<= (const char *s) const |
BString | operator+ (const BString &s) const |
BString | operator+= (const BString &s) |
BString | operator+ (BChar ch) const |
void | debugPrint () |
Static Public Member Functions | |
static BString | convert (Bool v) |
Converts Bool to string. More... | |
static BString | convert (BInt8 v) |
Converts Int8 to string. More... | |
static BString | convert (BUInt8 v) |
Converts UInt8 to string. More... | |
static BString | convert (BInt16 v) |
Converts Int16 to string. More... | |
static BString | convert (BUInt16 v) |
Converts UInt16 to string. More... | |
static BString | convert (BInt32 value) |
Converts int to string. More... | |
static BString | convert (BUInt32 value) |
Converts uint to string. More... | |
static BString | convert (double value, int eFormat=0) |
Converts double to string. More... | |
static BString | convert (BUInt64 value) |
Converts long long to string. More... | |
static BString | convert (BChar value) |
Converts BChar to string. More... | |
static BString | convertHex (BInt value) |
Converts int to string as hex value. More... | |
static BString | convertHex (BUInt value) |
Converts uint to string as hex value. More... | |
Protected Attributes | |
BRefString | orefs |
Constructor & Destructor Documentation
◆ BString() [1/4]
BString::BString | ( | const char * | str = 0 , |
int | len = -1 , | ||
Bool | copy = 0 | ||
) |
◆ BString() [2/4]
BString::BString | ( | const BString & | string | ) |
◆ BString() [3/4]
BString::BString | ( | BChar | c | ) |
◆ BString() [4/4]
BString::BString | ( | BInt | v | ) |
◆ ~BString()
BString::~BString | ( | ) |
Member Function Documentation
◆ add()
◆ append()
void BString::append | ( | const BString & | str | ) |
Append a string.
◆ clear()
void BString::clear | ( | ) |
Clear the string.
◆ compare() [1/2]
int BString::compare | ( | const BString & | string | ) | const |
Compare strings.
◆ compare() [2/2]
int BString::compare | ( | const char * | string | ) | const |
Compare strings.
◆ convert() [1/10]
◆ convert() [2/10]
◆ convert() [3/10]
◆ convert() [4/10]
◆ convert() [5/10]
◆ convert() [6/10]
◆ convert() [7/10]
◆ convert() [8/10]
| static |
Converts double to string.
◆ convert() [9/10]
◆ convert() [10/10]
◆ convertHex() [1/2]
◆ convertHex() [2/2]
◆ copy()
BString BString::copy | ( | ) | const |
Return an independant copy.
◆ debugPrint()
void BString::debugPrint | ( | ) |
◆ del()
void BString::del | ( | BUInt | start, |
int | len | ||
) |
Delete substring.
◆ find() [1/2]
int BString::find | ( | char | ch | ) | const |
Find ch in string searching forwards.
◆ find() [2/2]
int BString::find | ( | BString | str | ) | const |
Find string in string searching forwards.
◆ findReverse()
int BString::findReverse | ( | char | ch | ) | const |
Find ch in string searching backwards.
◆ hash()
BUInt32 BString::hash | ( | ) | const |
retunr a hash value
◆ insert()
◆ len()
BUInt BString::len | ( | ) | const |
Length of string.
◆ operator const char *()
| inline |
◆ operator!=() [1/2]
| inline |
◆ operator!=() [2/2]
| inline |
◆ operator+() [1/2]
◆ operator+() [2/2]
◆ operator+=()
◆ operator<() [1/2]
| inline |
◆ operator<() [2/2]
| inline |
◆ operator<=() [1/2]
| inline |
◆ operator<=() [2/2]
| inline |
◆ operator=()
◆ operator==() [1/2]
| inline |
◆ operator==() [2/2]
| inline |
◆ operator>() [1/2]
| inline |
◆ operator>() [2/2]
| inline |
◆ operator>=() [1/2]
| inline |
◆ operator>=() [2/2]
| inline |
◆ operator[]()
char & BString::operator[] | ( | int | pos | ) |
◆ pad()
◆ printf()
BString & BString::printf | ( | const char * | fmt, |
... | |||
) |
Formated print into the string.
◆ pullToken()
◆ removeSeparators()
Remove any char from sepatators from string.
◆ retFloat64()
BFloat64 BString::retFloat64 | ( | ) | const |
Return string as a double.
◆ retInt()
BInt BString::retInt | ( | ) | const |
Return string as a int.
◆ retStr()
const char * BString::retStr | ( | ) | const |
Ptr to char* representation.
◆ retUInt()
BUInt BString::retUInt | ( | ) | const |
Return string as a int.
◆ reverse()
BString BString::reverse | ( | ) | const |
Reverse character order.
◆ split()
Split string into an array based on the character separator.
◆ str()
const char * BString::str | ( | ) | const |
Ptr to char* representation.
◆ subString()
◆ toLower()
BString & BString::toLower | ( | ) |
Convert to lowercase.
◆ toUpper()
BString & BString::toUpper | ( | ) |
Convert to uppercase.
◆ truncate()
Member Data Documentation
◆ orefs
| protected |
The documentation for this class was generated from the following files:
Generated by
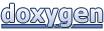